Enhancing Performance of Apache Spark Queries: Proven Strategies
Written on
Chapter 1: Introduction to Spark Query Optimization
Apache Spark is an efficient distributed computing framework designed to handle substantial datasets in a fault-tolerant and parallel manner. Yet, as data volumes increase, the execution speed of Spark queries can decline. This article delves into various strategies that can enhance the performance of your Spark queries.
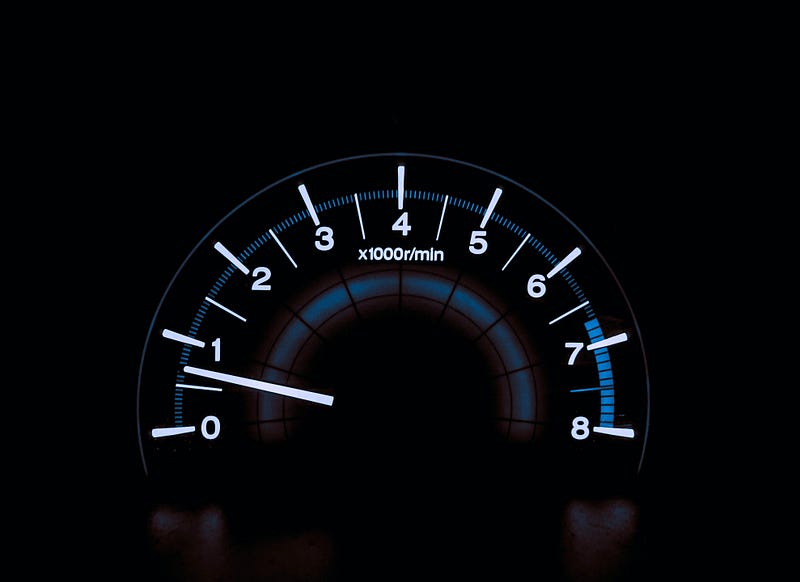
Section 1.1: Data Partitioning
When data is ingested into Spark, it is split into partitions spread across the cluster. By default, Spark partitions data according to the number of available cores, which may not be the most effective approach for your specific queries. By strategically partitioning your data, you can significantly enhance query performance.
For instance, if you have a vast dataset partitioned by date and frequently run queries filtering by this date, aligning your data partitions with your query patterns can minimize the data processed. You can partition your data in Spark using the repartition() or coalesce() methods. Here’s an example:
partitioned_data = raw_data.repartition(100, "date")
This command will create 100 partitions based on the date column.
Section 1.2: Data Compression
Implementing data compression can minimize the I/O operations needed to access your data, thus improving performance. Spark supports several compression codecs, such as Gzip, Snappy, and LZO. You can specify the compression codec when saving your data to disk, as shown below:
compressed_data = raw_data.write.option("compression", "snappy").parquet("path/to/compressedData")
This saves the data on disk using the Snappy compression codec.
Section 1.3: Caching Data
Storing frequently accessed data in memory can decrease the time spent retrieving it from disk, thereby enhancing performance. Spark offers various caching options, including cache() and persist(). For instance:
cached_data = raw_data.cache()
This caches the data in memory.
Section 1.4: Early Filtering
Applying filters early in the query can prevent unnecessary data processing, leading to improved performance. For example, rather than filtering data after loading it into Spark, you can apply the filter during the loading phase:
filtered_data = spark.read.parquet("path/to/data").filter("column == 'value'")
This filters the data before it is loaded into Spark.
Section 1.5: Broadcasting Small Tables
Broadcasting can enhance Spark application performance, particularly when joining a small table with a larger one. The concept involves disseminating the smaller table across all cluster nodes, allowing local joins without extensive data shuffling.
Here’s how to implement broadcasting in Python:
# Load the lookup table as a dictionary lookup_table = spark.read.csv("path/to/lookupTable")
.rdd
.map(lambda row: (row[0], row[1]))
.collectAsMap()
# Broadcast the lookup table to all nodes broadcast_table = spark.sparkContext.broadcast(lookup_table)
# Join the data with the broadcasted lookup table joined_data = raw_data.rdd
.map(lambda row: (row[0], row))
.mapValues(lambda row, lookup_table=broadcast_table.value: (row, lookup_table.get(row[0], "")))
.values()
In this example, we load the lookup table into a dictionary and broadcast it across the cluster, allowing efficient local joins.
Chapter 2: File Formats and Resource Management
Section 2.1: Choosing the Right File Format
The selection of file format can significantly affect performance. For instance, Parquet is a columnar format optimized for analytical tasks. Its design reduces I/O and enhances compression, improving query performance. To save data in Parquet format, use:
parquet_data = raw_data.write.parquet("path/to/parquetData")
Section 2.2: Minimizing Shuffling
Shuffling is an expensive operation in Spark, so it's essential to limit the amount of data shuffled. For example, when grouping data, consider grouping only a relevant subset:
grouped_data = raw_data
.rdd
.map(lambda row: (row[0], row))
.groupByKey()
.flatMapValues(lambda rows: rows)
Section 2.3: Optimizing Resource Allocation
Ensure that you allocate resources, such as memory and CPU, appropriately for your tasks. Spark allows you to configure resource usage based on your workload. For instance, to increase memory allocation, set the spark.executor.memory parameter:
conf = SparkConf().setAppName("myApp").set("spark.executor.memory", "8g") spark = SparkSession.builder.config(conf=conf).getOrCreate()
This allocates 8 GB of memory to each Spark executor.
Section 2.4: Using Efficient Transformations
Utilizing efficient built-in transformations can optimize performance. For example, instead of applying map() followed by filter(), consider reversing this order:
filtered_data = raw_data.filter("column == 'value'").map(lambda row: (row[0], row))
This approach filters the data before mapping it.
Section 2.5: Staying Updated with Spark Versions
Spark is continuously updated, with newer versions often featuring performance enhancements and bug fixes. Always ensure you are working with the latest version of Spark to maximize query performance.
In summary, numerous strategies exist to enhance the performance of Spark queries. By refining data partitioning, employing data compression, caching effectively, filtering early, utilizing broadcasting, selecting appropriate file formats, minimizing shuffling, optimizing resource allocation, employing efficient transformations, and keeping Spark up to date, you can significantly boost the efficiency of your Spark queries and handle large datasets more effectively.
Explore how to supercharge your Apache Spark queries using the Spark UI SQL tab in this informative video.
Learn about adaptive query execution techniques to speed up Spark SQL at runtime in this insightful video.