Enhancing Phishing URL Detection Using Python and APIs
Written on
Chapter 1: Understanding Phishing Attacks
Phishing is a deceptive tactic employed by cybercriminals to acquire sensitive data, such as credit card numbers or login credentials, by impersonating a trustworthy website.
Typically, in a phishing scheme, an unsuspecting user clicks on a compromised link that appears to lead to a legitimate site. When the victim inputs their credentials, these details are captured by the attackers, leading to unauthorized access to their accounts.
To combat this, we will utilize the IPQualityScore API, which is designed to identify fraudulent URLs. The initial step involves registering for the service to obtain your access key.
Section 1.1: Utilizing the IPQualityScore API
The Malicious URL Scanner API from IPQualityScore scans links in real-time, helping to identify phishing URLs, malware, and other suspicious links. It provides immediate risk assessments, enabling accurate detection of potentially harmful domains.
To safeguard your application, you can seamlessly integrate this API into your platform, allowing for real-time scanning without the hassle of false positives or missed threats.
Required Packages
To implement this solution, we will rely on just two libraries: requests for sending HTTP requests to the API, and urllib for URL encoding. The json module will also be necessary to handle API responses.
If you haven't installed the requests library yet, you can do so using pip:
pip install requests
Main Program
The core of our program will be straightforward:
import requests
import urllib
import json
url = "www.google.com" # Example URL
encoded_url = urllib.parse.quote(url, safe='')
data = requests.get(api_url + encoded_url)
print(json.dumps(data.json(), indent=4))
This code will produce an output similar to the following:
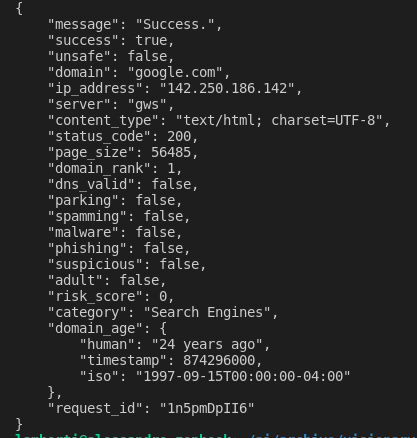
Section 1.2: Interpreting API Response
The output will include several fields, each providing crucial information about the scanned URL:
- domain: The final destination URL's domain after following any redirects.
- IP_address: The server's IP address associated with the domain.
- risk_score: An estimate of the likelihood that the URL is malicious, with scores of 85+ indicating high risk.
- suspicious: Indicates if the URL is suspected of malicious activity.
- phishing: Denotes if the URL is linked to phishing attempts.
- malware: Indicates if the URL is associated with malware.
- spamming: Indicates if the domain is connected to abusive email practices.
- adult: Signals whether the site hosts adult content.
Chapter 2: Practical Insights and Conclusion
The video titled "18 - End-to-End Machine Learning Project - Phishing Detection - Develop & Deploy ML app in Streamlit" provides a comprehensive guide on creating a phishing detection application using machine learning techniques.
In the second video, "Detection of Phishing Websites Using Machine Learning | Python Final Year IEEE Project 2023," you will find an in-depth exploration of machine learning methods for identifying phishing websites.
Based on my personal experience with this API, I've observed that legitimate sites with limited traffic or those that are relatively new may sometimes register a slightly elevated risk score.
Therefore, it's advisable to adjust your classification parameters according to the various fields provided to tailor the solution to your specific requirements.
If you found this content helpful, consider following me on Medium and visiting my website: alessandroai.com. You can also subscribe to the Artificialis newsletter here.