Enhancing Your React App with Box Plots Using the Visx Library
Written on
Chapter 1: Introduction to Visx
In this guide, we will explore how to seamlessly integrate box plots into your React application utilizing the Visx library. This powerful tool simplifies the process of adding visual elements to your projects.
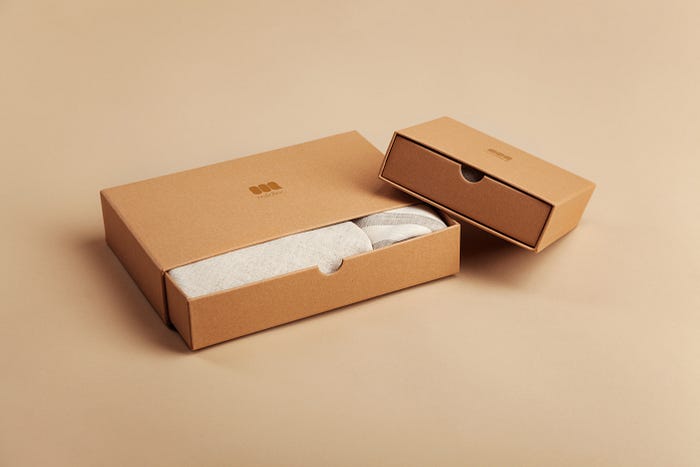
Section 1.1: Setting Up Your Environment
To begin, we need to install several essential packages. Use the following command to set everything up:
npm install @visx/gradient @visx/group @visx/mock-data @visx/pattern @visx/responsive @visx/scale @visx/stats @visx/tooltip
Section 1.2: Implementing Box Plots
Now that the packages are installed, we can proceed to implement box plots. Below is an example of how to do this:
import React from "react";
import { Group } from "@visx/group";
import { ViolinPlot, BoxPlot } from "@visx/stats";
import { LinearGradient } from "@visx/gradient";
import { scaleBand, scaleLinear } from "@visx/scale";
import genStats from "@visx/mock-data/lib/generators/genStats";
import {
Tooltip,
defaultStyles as defaultTooltipStyles,
useTooltip
} from "@visx/tooltip";
import { PatternLines } from "@visx/pattern";
const data = genStats(5);
// Define your accessor functions
const x = (d) => d.boxPlot.x;
const min = (d) => d.boxPlot.min;
const max = (d) => d.boxPlot.max;
const median = (d) => d.boxPlot.median;
const firstQuartile = (d) => d.boxPlot.firstQuartile;
const thirdQuartile = (d) => d.boxPlot.thirdQuartile;
const outliers = (d) => d.boxPlot.outliers;
const Example = ({ width, height }) => {
const {
tooltipOpen,
tooltipLeft,
tooltipTop,
tooltipData,
hideTooltip,
showTooltip
} = useTooltip();
const xMax = width;
const yMax = height - 120;
const xScale = scaleBand({
range: [0, xMax],
round: true,
domain: data.map(x),
padding: 0.4
});
const values = data.reduce((allValues, { boxPlot }) => {
allValues.push(boxPlot.min, boxPlot.max);
return allValues;
}, []);
const minYValue = Math.min(...values);
const maxYValue = Math.max(...values);
const yScale = scaleLinear({
range: [yMax, 0],
round: true,
domain: [minYValue, maxYValue]
});
const boxWidth = xScale.bandwidth();
const constrainedWidth = Math.min(40, boxWidth);
return width < 10 ? null : (
<Group top={20}>
{data.map((d, i) => (
<BoxPlot
key={boxplot-${i}}
data={d.boxPlot}
x={xScale(x(d))}
width={constrainedWidth}
yScale={yScale}
{...{
minProps: {
onMouseOver: () => {
showTooltip({
tooltipTop: yScale(min(d)) + 40,
tooltipLeft: xScale(x(d)) + constrainedWidth + 5,
tooltipData: { min: min(d), name: x(d) }
});
},
onMouseLeave: hideTooltip
},
maxProps: {
onMouseOver: () => {
showTooltip({
tooltipTop: yScale(max(d)) + 40,
tooltipLeft: xScale(x(d)) + constrainedWidth + 5,
tooltipData: { max: max(d), name: x(d) }
});
},
onMouseLeave: hideTooltip
},
boxProps: {
onMouseOver: () => {
showTooltip({
tooltipTop: yScale(median(d)) + 40,
tooltipLeft: xScale(x(d)) + constrainedWidth + 5,
tooltipData: { ...d.boxPlot, name: x(d) }
});
},
onMouseLeave: hideTooltip
},
medianProps: {
style: { stroke: "white" },
onMouseOver: () => {
showTooltip({
tooltipTop: yScale(median(d)) + 40,
tooltipLeft: xScale(x(d)) + constrainedWidth + 5,
tooltipData: { median: median(d), name: x(d) }
});
},
onMouseLeave: hideTooltip
}
}}
/>
))}
{tooltipOpen && tooltipData && (
<Tooltip
top={tooltipTop}
left={tooltipLeft}
style={defaultTooltipStyles}
>
{tooltipData.name}
{tooltipData.max && Max: ${tooltipData.max}}
{tooltipData.thirdQuartile && Third Quartile: ${tooltipData.thirdQuartile}}
{tooltipData.median && Median: ${tooltipData.median}}
{tooltipData.firstQuartile && First Quartile: ${tooltipData.firstQuartile}}
{tooltipData.min && Min: ${tooltipData.min}}
</Tooltip>
)}
</Group>
);
};
export default function App() {
return (
<svg width={800} height={500}>
<Example width={800} height={500} /></svg>
);
}
The data variable contains the information for the box plot, while the functions that follow serve as accessors for various statistical values. In the Example component, we utilize the useTooltip hook to display tooltips when hovering over the box plots.
We collect the necessary values for the box plots and establish a scale for the y-axis. The box plots are rendered using data.map, which creates box plot components for each data point. The ViolinPlot component is used to display the surrounding violin plot, while the BoxPlot component creates the box plot itself.
Tooltips are displayed upon hovering over different parts of the box plot, providing detailed information about the data.
Chapter 2: Conclusion
In conclusion, integrating box and violin plots into your React application is straightforward with the Visx library. This approach not only enhances the visual appeal of your data presentation but also improves user interaction and understanding of the data.
Explore more about creating effective visualizations with D3, React, and Typescript in the video titled "Data Visualization with D3, React, visx and Typescript: 11 - Creating a Bar Chart with visx."
Learn how to create a useful chart with visx in the video titled "Data Visualization with D3, React, visx and Typescript: 12 - Creating a Useful Chart with visx."