Getting Started with SQLite in Python on Windows
Written on
Introduction to SQLite
SQLite is a compact, file-based relational database management system (RDBMS) that enjoys widespread popularity due to its straightforward nature, user-friendliness, and lack of configuration requirements. This serverless, self-contained database engine makes it particularly suitable for embedded systems, mobile apps, and small to medium-sized projects.
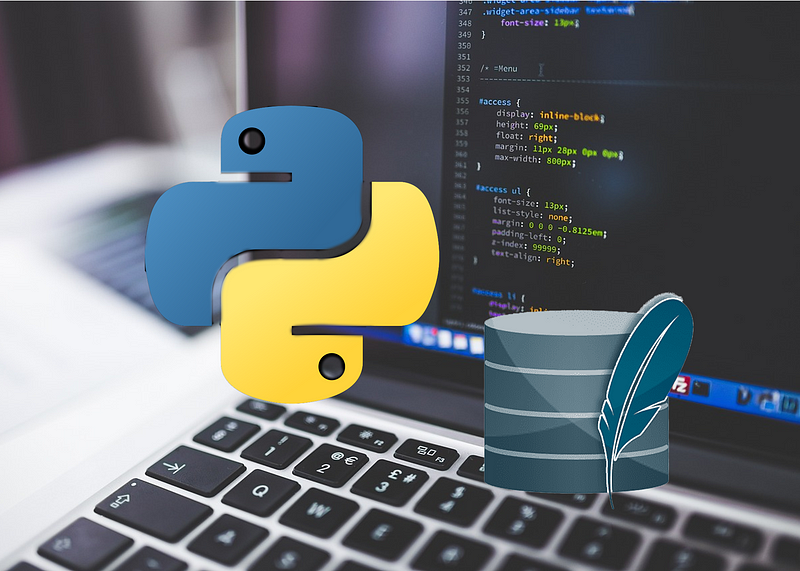
What is SQLite?
SQLite is an open-source C library that delivers a lightweight, disk-based database without the need for a separate server process. It functions as a self-contained, serverless, and zero-configuration database engine, which means that it does not need a dedicated server to operate, and the entire database is stored in a single file.
Benefits of Using SQLite
- User-Friendly: SQLite is renowned for its ease of use. Setting up a database is simple and does not require complex configurations or extensive administration.
- Serverless: Unlike conventional database systems such as MySQL or PostgreSQL, SQLite does not depend on an external server process, making it ideal for smaller projects where a dedicated server might be unnecessary.
- Zero Configuration: With SQLite, you can get started immediately without the hassle of server configurations or user management.
- Cross-Platform: SQLite is compatible with various operating systems, including Windows, Linux, and macOS, making it a versatile choice for projects that need to function across different platforms.
- Embeddable: Its small size and simplicity make SQLite a popular choice for embedded systems and mobile applications, allowing it to be integrated directly into applications without requiring a separate server.
Using SQLite with Python on Windows
Python comes with a built-in module called sqlite3, which offers a straightforward interface for working with SQLite databases. Below is a step-by-step guide for using SQLite in Python on a Windows platform:
Note: SQLite is usually pre-installed on Windows. Simply import it and start using it. If you encounter any issues, consider searching online for solutions.
Creating a Database and Establishing a Connection
Use the sqlite3 module to create a database file and connect to it using Python.
Performing CRUD Operations
Utilize SQL commands to execute Create, Read, Update, and Delete (CRUD) operations on your SQLite database.
Exception Handling
Implement error handling to address potential issues, such as connection failures or SQL command errors.
Sample Code — Creating a Database
import sqlite3
# Connect to a database (creates a new file if it doesn't exist)
conn = sqlite3.connect('example.db')
# Create a cursor object to execute SQL commands
cursor = conn.cursor()
# Execute a SQL command to create a table
cursor.execute('''
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
name TEXT NOT NULL,
age INTEGER
)
''')
# Insert data into the 'users' table
cursor.execute("INSERT INTO users (name, age) VALUES (?, ?)", ('John Doe', 25))
# Execute a SELECT query
cursor.execute("SELECT * FROM users")
# Fetch all results
results = cursor.fetchall()
# Print the results
for row in results:
print(row)
# Close the connection
conn.close()
After running the code, a database file named "example.db" will be created.
Inserting Data
# Connect to the database again
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# Insert data into the 'users' table
cursor.execute("INSERT INTO users (name, age) VALUES (?, ?)", ('John Doe', 25))
# Commit the changes and close the connection
conn.commit()
conn.close()
Querying Data
# Connect to the database
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# Execute a SELECT query
cursor.execute("SELECT * FROM users")
# Fetch all results
results = cursor.fetchall()
# Print the results
for row in results:
print(row)
# Close the connection
conn.close()
Updating & Deleting Data
# Connect to the database
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# Update data
cursor.execute("UPDATE users SET age=? WHERE name=?", (30, 'John Doe'))
# Delete data
cursor.execute("DELETE FROM users WHERE name=?", ('John Doe',))
# Commit the changes and close the connection
conn.commit()
conn.close()
Error Handling
try:
# Your database code here
except sqlite3.Error as e:
print("SQLite error:", e)
finally:
# Ensure the connection is closed
conn.close()
Where to Use SQLite?
Desktop Applications: SQLite serves as a fantastic option for desktop applications requiring a lightweight, embedded database. When developing a Windows desktop application using Python, SQLite offers seamless integration for local data storage.
Prototyping and Rapid Development: When you need to prototype or quickly develop an application, the overhead of setting up a full database server can be cumbersome. SQLite's serverless architecture allows developers to focus on building application logic without the burden of database management.
Educational Purposes: SQLite is an ideal platform for teaching or learning database concepts and SQL due to its simple setup and minimal administrative needs.
Project Ideas Using SQLite in Python on Windows
Here are some engaging project ideas that you can create with SQLite and Python:
- Task Manager: Develop a simple application that allows users to manage tasks, complete with adding, editing, and deleting functionalities.
- Personal Finance Tracker: Create an application to track income, expenses, and budgets, utilizing SQLite for transaction storage and report generation.
- Address Book: Design an address book application to store and manage contacts, using SQLite for data storage.
- Blog Platform: Build a basic blog platform where users can create, edit, and delete posts, with SQLite managing the content and metadata.
- Inventory Management System: Create a system to track products and stock levels, leveraging SQLite for product information and transaction history.
These project ideas range from simple to complex, providing various opportunities to explore different aspects of SQLite and Python development on Windows. Choose a project that resonates with your interests and skill level, and feel free to add your own creative flair to make it unique!
To further expand your knowledge on using SQLite with Python, check out the following videos:
This video provides a comprehensive course on SQLite databases with Python.
This tutorial offers a complete overview of creating databases, tables, and running queries using Python and SQLite.
Thank you for reading! If you found this information helpful, consider following me for more content.