Swap Nodes in Pairs: A Comprehensive Guide for Technical Interviews
Written on
Chapter 1: Understanding the Problem
When preparing for technical interviews, it's essential to grasp various algorithmic problems thoroughly. One such problem is to swap every two adjacent nodes in a linked list and return the modified list's head. Notably, this task must be accomplished without altering the node values themselves—only the nodes may be rearranged.
Example Cases:
Input: head = [1,2,3,4]
Output: [2,1,4,3]
Input: head = []
Output: []
Input: head = [1]
Output: [1]
Constraints: The total number of nodes can range from 0 to 100, and each node's value is bounded between 0 and 100.
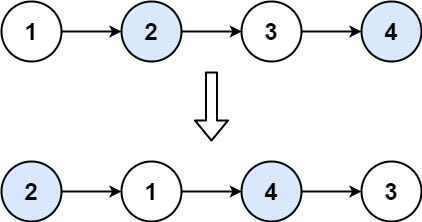
Chapter 2: Recursive Approach
Intuition Behind the Solution
This problem requires us to swap adjacent nodes rather than reversing the entire linked list. The strategy involves traversing the list in pairs using recursion, allowing us to swap nodes as we backtrack.
In each recursive call, we extract two nodes to swap and then pass the remaining nodes to the next recursive call. This is effective because a sub-list remains a linked list, making it compatible with our recursive approach. After each call returns the swapped sub-list, we swap the current two nodes and reconnect them to the result of the recursive call.
Algorithm Steps:
- Start recursion with the head node of the linked list.
- Each recursive call handles the swapping of a pair of nodes (firstNode and secondNode).
- Make a recursive call for the next pair, continuing until the end of the list is reached.
- Once backtracking occurs, swap the firstNode and secondNode, returning the new head (secondNode).
Python Implementation:
class Solution(object):
def swapPairs(self, head: ListNode) -> ListNode:
if not head or not head.next:
return headfirst_node = head
second_node = head.next
first_node.next = self.swapPairs(second_node.next)
second_node.next = first_node
return second_node
Java Implementation:
class Solution {
public ListNode swapPairs(ListNode head) {
if ((head == null) || (head.next == null)) {
return head;}
ListNode firstNode = head;
ListNode secondNode = head.next;
firstNode.next = swapPairs(secondNode.next);
secondNode.next = firstNode;
return secondNode;
}
}
Video Explanation: This video titled "Swap Nodes in Pairs - Apple Interview Question - Leetcode 24" provides a comprehensive walkthrough of solving this problem, illustrating the recursive approach in detail.
Chapter 3: Iterative Approach
The iterative method follows a similar logic but executes the swaps on-the-fly rather than through recursion. We traverse the list in pairs, linking nodes as we go.
Algorithm Steps:
- Use a dummy node as a precursor to facilitate operations on the head.
- As you iterate, identify pairs to swap (firstNode and secondNode).
- After a swap, link the previous node to the new head of the swapped pair.
- Update pointers accordingly to continue iterating through the list.
Python Implementation:
class Solution:
def swapPairs(self, head: ListNode) -> ListNode:
dummy = ListNode(-1)
dummy.next = head
prev_node = dummy
while head and head.next:
first_node = head
second_node = head.next
prev_node.next = second_node
first_node.next = second_node.next
second_node.next = first_node
prev_node = first_node
head = first_node.next
return dummy.next
Java Implementation:
class Solution {
public ListNode swapPairs(ListNode head) {
ListNode dummy = new ListNode(-1);
dummy.next = head;
ListNode prevNode = dummy;
while ((head != null) && (head.next != null)) {
ListNode firstNode = head;
ListNode secondNode = head.next;
prevNode.next = secondNode;
firstNode.next = secondNode.next;
secondNode.next = firstNode;
prevNode = firstNode;
head = firstNode.next; // jump
}
return dummy.next;
}
}
Video Explanation: This video titled "Swap Nodes in Pairs - Facebook Interview Question - LeetCode 24" further elaborates on the iterative approach to solving the same problem, providing additional insights into best practices.
Conclusion
With these two approaches—recursive and iterative—you are well-equipped to tackle the "Swap Nodes in Pairs" interview question effectively. Stay tuned for more intriguing algorithmic challenges and insights from the world of software engineering.
— I am a senior software engineer at MANNG. Follow me for more engaging interview content.